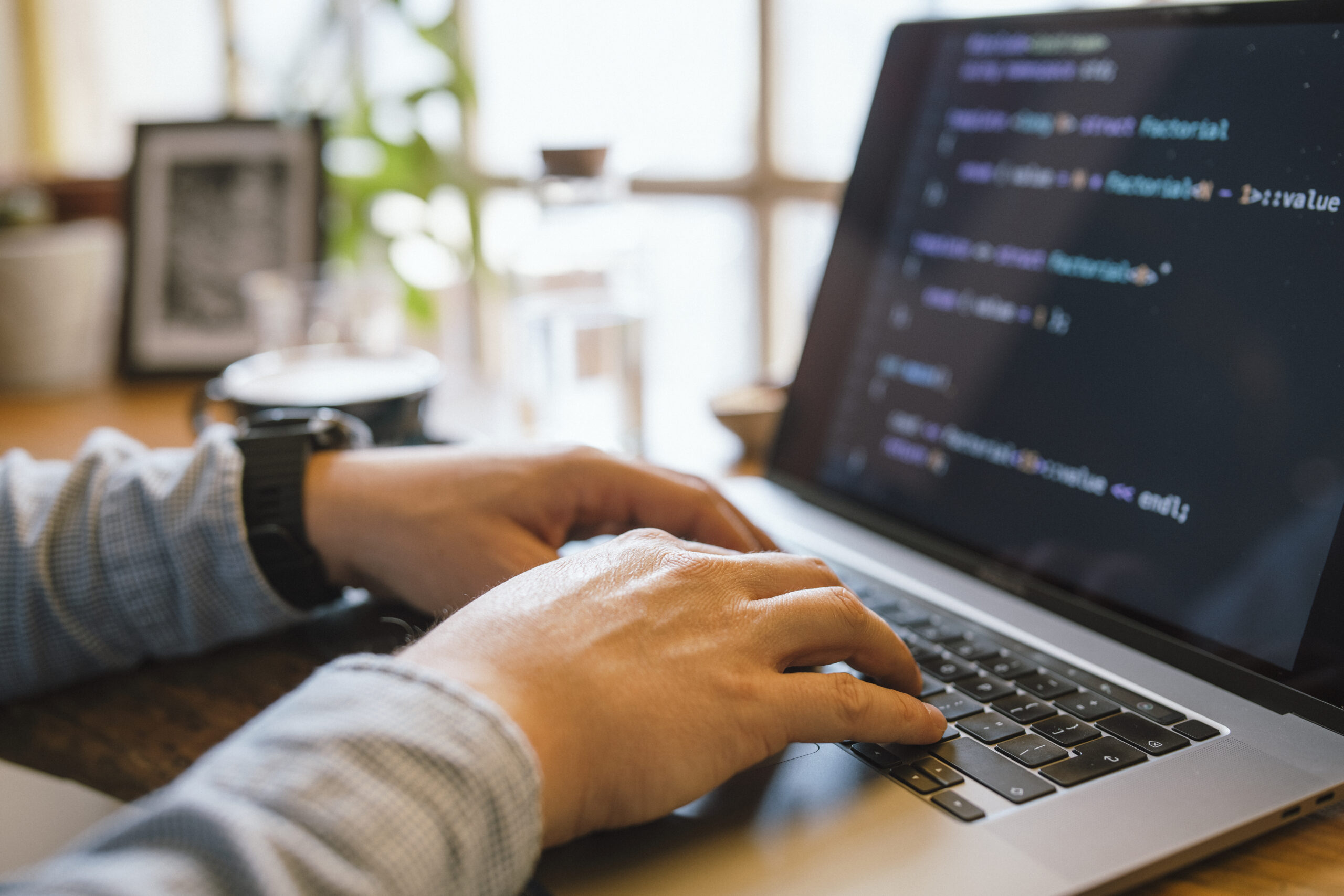
Debugging is Just about the most vital — nonetheless frequently disregarded — capabilities in a very developer’s toolkit. It isn't really pretty much correcting damaged code; it’s about comprehending how and why factors go Completely wrong, and Finding out to Assume methodically to solve difficulties successfully. Whether you're a beginner or simply a seasoned developer, sharpening your debugging skills can save hours of disappointment and substantially help your efficiency. Listed here are numerous techniques to help you developers degree up their debugging sport by me, Gustavo Woltmann.
Master Your Resources
On the list of fastest strategies developers can elevate their debugging expertise is by mastering the equipment they use on a daily basis. When producing code is just one Section of advancement, understanding how to connect with it properly in the course of execution is equally significant. Present day improvement environments occur Outfitted with potent debugging abilities — but a lot of developers only scratch the area of what these instruments can perform.
Consider, for example, an Built-in Advancement Environment (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These equipment allow you to established breakpoints, inspect the value of variables at runtime, action by means of code line by line, and also modify code on the fly. When applied appropriately, they let you notice precisely how your code behaves all through execution, and that is invaluable for monitoring down elusive bugs.
Browser developer tools, for instance Chrome DevTools, are indispensable for front-conclude builders. They help you inspect the DOM, keep track of community requests, view true-time overall performance metrics, and debug JavaScript from the browser. Mastering the console, resources, and network tabs can convert irritating UI troubles into workable tasks.
For backend or procedure-stage developers, equipment like GDB (GNU Debugger), Valgrind, or LLDB give deep Manage about running processes and memory management. Mastering these tools could have a steeper Mastering curve but pays off when debugging performance concerns, memory leaks, or segmentation faults.
Outside of your IDE or debugger, turn out to be cozy with Model Command systems like Git to know code historical past, come across the precise minute bugs were being introduced, and isolate problematic alterations.
In the long run, mastering your applications means going over and above default options and shortcuts — it’s about producing an personal expertise in your enhancement atmosphere to ensure when difficulties occur, you’re not shed at the hours of darkness. The better you recognize your applications, the greater time you could spend resolving the particular problem instead of fumbling via the process.
Reproduce the issue
Just about the most vital — and often overlooked — steps in effective debugging is reproducing the issue. Prior to leaping in the code or earning guesses, builders want to create a constant environment or state of affairs wherever the bug reliably appears. With out reproducibility, fixing a bug becomes a activity of probability, typically leading to squandered time and fragile code alterations.
Step one in reproducing a problem is accumulating as much context as possible. Talk to thoughts like: What actions led to The difficulty? Which surroundings was it in — improvement, staging, or output? Are there any logs, screenshots, or mistake messages? The more depth you've, the a lot easier it results in being to isolate the exact disorders beneath which the bug takes place.
As soon as you’ve collected plenty of info, endeavor to recreate the trouble in your neighborhood atmosphere. This may imply inputting the exact same information, simulating very similar user interactions, or mimicking technique states. If The difficulty appears intermittently, take into account writing automated assessments that replicate the sting circumstances or point out transitions involved. These exams not simply help expose the trouble but will also stop regressions Sooner or later.
In some cases, the issue could possibly be ecosystem-particular — it would materialize only on certain working programs, browsers, or less than certain configurations. Using instruments like Digital devices, containerization (e.g., Docker), or cross-browser testing platforms can be instrumental in replicating these types of bugs.
Reproducing the trouble isn’t simply a stage — it’s a mentality. It demands patience, observation, and a methodical tactic. But when you can continually recreate the bug, you are by now halfway to fixing it. That has a reproducible scenario, you can use your debugging instruments more properly, check opportunity fixes safely and securely, and talk additional clearly using your group or end users. It turns an abstract grievance right into a concrete obstacle — Which’s where by builders prosper.
Examine and Comprehend the Mistake Messages
Error messages are often the most useful clues a developer has when a little something goes Completely wrong. Rather than looking at them as discouraging interruptions, developers really should study to deal with error messages as immediate communications with the technique. They usually tell you exactly what transpired, the place it occurred, and sometimes even why it transpired — if you understand how to interpret them.
Begin by studying the information meticulously and in comprehensive. A lot of developers, specially when beneath time pressure, look at the very first line and straight away start off creating assumptions. But further inside the mistake stack or logs may possibly lie the real root cause. Don’t just duplicate and paste error messages into search engines — read through and comprehend them initially.
Break the mistake down into components. Could it be a syntax mistake, a runtime exception, or possibly a logic mistake? Does it place to a specific file and line range? What module or function activated it? These questions can information your investigation and point you toward the liable code.
It’s also useful to be aware of the terminology of the programming language or framework you’re employing. Mistake messages in languages like Python, JavaScript, or Java generally follow predictable designs, and Discovering to recognize these can drastically speed up your debugging approach.
Some faults are vague or generic, As well as in those circumstances, it’s very important to examine the context through which the mistake occurred. Examine relevant log entries, enter values, and up to date modifications while in the codebase.
Don’t overlook compiler or linter warnings either. These typically precede much larger problems and provide hints about probable bugs.
Ultimately, error messages usually are not your enemies—they’re your guides. Mastering to interpret them correctly turns chaos into clarity, assisting you pinpoint concerns more rapidly, lower debugging time, and turn into a additional economical and confident developer.
Use Logging Correctly
Logging is One of the more potent equipment in a very developer’s debugging toolkit. When employed proficiently, it provides actual-time insights into how an application behaves, supporting you fully grasp what’s going on under the hood with no need to pause execution or action through the code line by line.
A great logging method begins with figuring out what to log and at what amount. Common logging levels incorporate DEBUG, Data, WARN, ERROR, and FATAL. Use DEBUG for in depth diagnostic information all through advancement, Data for standard gatherings (like thriving commence-ups), WARN for prospective difficulties that don’t split the appliance, ERROR for real difficulties, and Deadly when the procedure can’t continue.
Stay clear of flooding your logs with excessive or irrelevant data. An excessive amount logging can obscure critical messages and slow down your procedure. Center on crucial occasions, point out alterations, input/output values, and significant selection details with your code.
Format your log messages Plainly and constantly. Consist of context, such as timestamps, ask for IDs, and function names, so it’s easier to trace difficulties in distributed systems or multi-threaded environments. Structured logging (e.g., JSON logs) might make it even simpler to parse and filter logs programmatically.
During debugging, logs Enable you to monitor how variables evolve, what circumstances are achieved, and what branches of logic are executed—all without having halting This system. They’re In particular important in output environments where by stepping by code isn’t feasible.
On top of that, use logging frameworks and equipment (like Log4j, Winston, or Python’s logging module) that support log rotation, filtering, and integration with monitoring dashboards.
Finally, smart logging is about equilibrium and clarity. Using a perfectly-imagined-out logging solution, you'll be able to lessen the time it requires to identify concerns, get deeper visibility into your programs, and Increase the overall maintainability and dependability within your code.
Believe Like a Detective
Debugging is not merely a complex task—it's a sort of investigation. To effectively establish and repair bugs, builders should tactic the process just like a detective solving a thriller. This mindset aids stop working elaborate issues into manageable components and stick to clues logically to uncover the basis lead to.
Start out by accumulating evidence. Consider the signs and symptoms of the situation: mistake messages, incorrect output, or general performance difficulties. Similar to a detective surveys against the law scene, collect as much appropriate information and facts as you'll be able to with out leaping to conclusions. Use logs, examination conditions, and consumer studies to piece collectively a clear picture of what’s occurring.
Subsequent, variety hypotheses. Ask yourself: What can be resulting in this behavior? Have any improvements not long ago been created on the codebase? Has this difficulty happened just before less than comparable conditions? The objective should be to slim down opportunities and identify opportunity culprits.
Then, test your theories systematically. Make an effort to recreate the situation in a very managed ecosystem. If you suspect a specific operate or part, isolate it and confirm if the issue persists. Similar to a detective conducting interviews, check with your code inquiries and Allow the results guide you nearer to the reality.
Fork out shut focus to little information. Bugs normally hide during the the very least predicted areas—like a missing semicolon, an off-by-one mistake, or simply a race issue. Be comprehensive and affected individual, resisting the urge to patch The problem with out absolutely comprehension it. Temporary fixes may cover the real trouble, just for it to resurface later.
Lastly, retain notes on Everything you tried and discovered. Equally as detectives log their investigations, documenting your debugging system can help save time for potential problems and assistance Many others realize your reasoning.
By wondering like a detective, builders can sharpen their analytical competencies, strategy problems methodically, and grow to be more practical at uncovering hidden problems in advanced devices.
Publish Checks
Creating assessments is among the most effective strategies to boost your debugging techniques and overall advancement effectiveness. Assessments don't just help catch bugs early and also function a safety Internet that offers you self-assurance when building changes for your codebase. A effectively-examined software is much easier to debug mainly because it allows you to pinpoint specifically the place and when a challenge takes place.
Start with unit tests, which concentrate on personal functions or modules. These tiny, isolated assessments can promptly expose whether a specific piece of logic is working as anticipated. Each time a examination fails, you straight away know where to search, substantially minimizing time invested debugging. Unit checks are Primarily handy for catching regression bugs—troubles that reappear soon after Earlier getting set.
Next, combine integration assessments and conclude-to-stop tests into your workflow. These help ensure that many portions of your application operate collectively easily. They’re especially useful for catching bugs that manifest in elaborate programs with numerous components or products and services interacting. If a little something breaks, your checks can inform you which Portion of the pipeline failed and underneath what problems.
Creating tests also forces you to definitely Consider critically about your code. To check a feature thoroughly, you may need to know its inputs, envisioned outputs, and edge circumstances. This volume of being familiar with In a natural way leads to higher code composition and less bugs.
When debugging an issue, producing a failing check that reproduces the bug can be a strong starting point. After the test fails continually, you'll be able to deal with fixing the bug and look at your check move when the issue is fixed. This method makes certain that precisely the same bug doesn’t return in the future.
Briefly, crafting exams turns debugging from the irritating guessing video game into a structured and predictable method—serving to you capture much more bugs, more rapidly plus more reliably.
Take Breaks
When debugging a difficult situation, it’s quick to become immersed in the trouble—observing your monitor for hours, making an attempt Option just after Answer. But Among the most underrated debugging applications is simply stepping away. Taking breaks assists you reset your brain, minimize disappointment, and infrequently see the issue from a new point of view.
When you are too close to the code for as well extended, cognitive tiredness sets in. You may perhaps get started overlooking noticeable errors or misreading code that you wrote just hours earlier. Within this state, your Mind results in being significantly less productive at dilemma-fixing. A short walk, a espresso split, as well as switching to a distinct activity for 10–15 minutes can refresh your focus. Several developers report locating the basis of a challenge once they've taken time and energy to disconnect, allowing their subconscious perform within the background.
Breaks also assistance protect against burnout, Specially for the duration of extended debugging periods. Sitting in front of a display, mentally caught, is not just unproductive but additionally draining. Stepping away permits you to return with renewed Power and a clearer mindset. You may perhaps quickly detect a missing semicolon, a logic flaw, or perhaps a misplaced variable that eluded you just before.
In the event you’re stuck, a very good general guideline should be to established a timer—debug actively for 45–sixty minutes, then have a 5–ten minute crack. Use that point to move all over, extend, or do one thing unrelated to code. It may sense counterintuitive, In particular beneath tight deadlines, but it surely in fact leads to speedier and simpler debugging Ultimately.
In a nutshell, having breaks is just not a sign of weak point—it’s a sensible tactic. It provides your Mind Room to breathe, increases your viewpoint, and assists you steer clear of the tunnel vision that often blocks your development. Debugging is actually a psychological puzzle, and relaxation is a component of solving it.
Study From Every single Bug
Every bug you come across is a lot more than just a temporary setback—It can be a possibility to develop being a developer. Whether it’s a syntax error, a logic flaw, or possibly a deep architectural difficulty, each one can educate you a little something valuable should you make the effort to replicate and analyze what went Improper.
Start out by inquiring your self several essential issues as soon as the bug is fixed: What caused it? Why did it go unnoticed? Could it are already caught previously with better methods like unit tests, code assessments, or logging? The solutions frequently expose blind spots within your workflow or comprehending and assist you Develop more robust coding routines going forward.
Documenting bugs can also be an outstanding pattern. Continue to keep a developer journal or manage a log in which you Take note down bugs you’ve encountered, the way you solved them, and Anything you learned. Over time, you’ll start to see styles—recurring challenges or popular faults—you can proactively prevent.
In staff environments, sharing Whatever you've realized from a bug with your friends is often In particular strong. No matter if it’s via a Slack concept, a short generate-up, or A fast expertise-sharing Gustavo Woltmann coding session, aiding Other people avoid the same challenge boosts crew efficiency and cultivates a stronger Discovering lifestyle.
Much more importantly, viewing bugs as classes shifts your frame of mind from disappointment to curiosity. Rather than dreading bugs, you’ll start appreciating them as critical portions of your improvement journey. After all, many of the greatest developers are certainly not the ones who produce excellent code, but those who repeatedly discover from their issues.
Ultimately, Each individual bug you correct provides a fresh layer towards your ability established. So subsequent time you squash a bug, have a moment to mirror—you’ll occur away a smarter, additional capable developer on account of it.
Summary
Bettering your debugging competencies will take time, exercise, and patience — although the payoff is large. It helps make you a far more economical, assured, and capable developer. Another time you're knee-deep within a mysterious bug, recall: debugging isn’t a chore — it’s an opportunity to become greater at Anything you do.